Vb.Net Programmes
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles Button1.Click
Dim i As Integer
Dim week(6) As String week(0) = "Sunday" week(1) = "Monday" week(2) = "Tuesday" week(3) = "Wednesday" week(4) = "Thursday" week(5) = "Friday" week(6) = "Saturday"
For i = 0 To week.Length - 1
MsgBox(week(i))
Next
End Sub
End Class

Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Dim array As Integer() = {10, 30, 50}
For Each element As Integer In array
MsgBox(element)
Next
End Sub
End Class
x = 10
If (x Mod 2) = 0 Then
Console.WriteLine("x is an even number")
Else
Console.WriteLine("x is an odd number")
End If
Console.ReadLine()
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles Button1.Click
Dim i As Integer
Dim week(6) As String week(0) = "Sunday" week(1) = "Monday" week(2) = "Tuesday" week(3) = "Wednesday" week(4) = "Thursday" week(5) = "Friday" week(6) = "Saturday"
For i = 0 To week.Length - 1
MsgBox(week(i))
Next
End Sub
End Class
CheckBox Control

Protected Sub Button1_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles Button1.Click
If CheckBox1.Checked = True Then
Literal1.Text = "You Checked the Box"
Else
Literal1.Text = "You Did Not Check the Box"
End If
End Sub
Public Class Form1 Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click Dim msg As String = "" If CheckBox1.Checked = True Then msg = "net-informations.com" End If If CheckBox2.Checked = True Then msg = msg & " vb.net-informations.com" End If If CheckBox3.Checked = True Then msg = msg & " csharp.net-informations.com" End If If msg.Length > 0 Then MsgBox(msg & " selected ") Else MsgBox("No checkbox selected") End If CheckBox1.ThreeState = True End Sub
Multi-Dimensional Array: VB.Net मे कई dimensions के array create किए जा सकते हैं। ऐसे arrayजिसमे एक से जायदा subscripts होती हैं उन्हे multi-dimensional array कहते हैं। MD array कोdeclare करने के लिए भी Dim, private या public statement का use किया जाता है। इसे declare करते समय इसमे एक से ज्यादा subscripts दी जाती हैं। यह subscripts के multiple के बराबर values storeकर सकते हैं जैसे n(2,3) array मे 6 values store की जा सकती हैं।
Syntax:Dim <array name> (script1, script2 ...) As <Data Type>Exp-Dim matrix (3, 3) as IntegerDim T (2, 3, 4, 2) As DoubleInitializing Multi-Dimensional Array:MD Array को initialize करने के लिए Carly braces का use करते हैं।Dim n (3, 3) As Integer = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e AsSystem.EventArgs) Handles Button1.Click
Dim mat(3, 3) As Integer
Dim i, j As Integer
'Take Input
For i = 0 To 2
For j = 0 To 2
mat(i, j) = InputBox("Enter value on Matrix")
Next
Next
'Printing Matrix
Label1.Text = ""
For i = 0 To 2
For j = 0 To 2
Label1.Text = Label1.Text & " " & mat(i, j)
Next
Label1.Text = Label1.Text & Chr(13)
Next
End Sub
End Class
How to Use ForEach loop with Arrays ?
Public Class Form1Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Dim array As Integer() = {10, 30, 50}
For Each element As Integer In array
MsgBox(element)
Next
End Sub
End Class
Optional Arguments:
VB.Net मे optional arugments भी दिये जा सकते है। optional arguments देने के लिए argument को declareकरते समय Byval/ Byref keyword के पहले Optional keyword का use किया जाता है और इसमे default value दी जाती है। Default value देना compulsory होता है। जिसका syntax निम्न है।
[Optional] [Byval / ByRef] <variable_name> As <Data Type> = <Default Value>
Ex-
Private Function Sum(ByVal a As Integer, Optional ByVal b As Integer = 10) AsInteger
Return (a + b)
End Function
Public Class Form1 Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click Dim totalMarks As Integer totalMarks = 59 If totalMarks >= 80 Then MsgBox("Gor Higher First Class ") ElseIf totalMarks >= 60 Then MsgBox("Gor First Class ") ElseIf totalMarks >= 40 Then MsgBox("Just pass only") Else MsgBox("Failed") End If End Sub End Class
Dim x As IntegerPublic Class Form1 Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click Dim totalMarks As Integer totalMarks = 39 If totalMarks >= 50 Then MsgBox("passed ") Else MsgBox("Failed ") End Sub End Class
x = 10
If (x Mod 2) = 0 Then
Console.WriteLine("x is an even number")
Else
Console.WriteLine("x is an odd number")
End If
Console.ReadLine()
End Sub
Public Class Form1 Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click Dim var As Integer Dim startVal As Integer Dim endVal As Integer startVal = 1 endVal = 5 For var = startVal To endVal MsgBox("Message Box Shows " & var & " Times ") Next var End Sub End Class
Public Class Form1 Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click Dim counter As Integer counter = 1 While (counter <= 10) MsgBox("Counter Now is : " counter) counter = counter + 1 End While End Sub
If….Then…ElseIf Statement
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click Dim Mark As Integer Dim Grade as String Mark = TextBox1.Text If myNumber >=80 Then Grade="A" ElseIf Mark>=60 and Mark<80 then Grade="B" ElseIf Mark>=40 and Mark<60 then Grade="C" Else Grade="D" End If End SubSelect Case
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click 'Examination Marks Dim mark As Single mark = TextBox1.Text Select Case mark Case 0 To 49 Label1.Text = "E" Case 50 To 59 Label1.Text = "D" Case 60 To 69 Label1.Text = "C" Case 70 To 79 Label1.Text = "B" Case 80 To 100 Label1.Text = "A" Case Else Label1.Text = "Error, please reenter the mark" End Select End Sub
For….Next Loop
Dim counter as Integer For counter=1 to 10 ListBox1.Items.Add (counter) Next* The program will enter number 1 to 10 into the list box.
Dim counter , sum As Integer
For counter=1 to 100 step 10
sum+=counter
ListBox1.Items.Add (sum)
Next
* The program will calculate the sum of the numbers as follows:
sum=0+10+20+30+40+……
Dim counter, sum As Integer
sum = 1000
For counter = 100 To 5 Step -5
sum - = counter
ListBox1.Items.Add(sum)
Next
*Notice that increment can be negative.
The program will compute the
subtraction as follow:
1000-100-95-90-……….
Dim n as Integer
For n=1 to 10
If n>6 then
Exit For
End If
Else
ListBox1.Items.Add ( n)
Next
The process will stop when n is greater than 6.
Timer Control
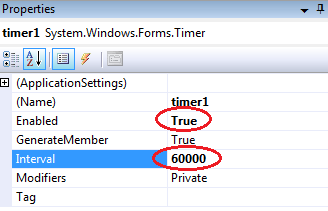
Public Class Form1
Dim second As Integer
Private Sub Form1_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
Timer1.Interval = 1000
Timer1.Start() 'Timer starts functioning
End Sub
Private Sub Timer1_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Timer1.Tick
Label1.Text = DateTime.Now.ToString
second = second + 1
If second >= 10 Then
Timer1.Stop() 'Timer stops functioning
MsgBox("Timer Stopped....")
End If
End Sub
End Class
Comments
Post a Comment